在AI如火如荼的当下,WordPress集成AI功能可以显著提升网站的内容生成、用户体验和安全性。首先可以通过多种AI插件来集成AI功能,但这不是我们要讲的,我们要从基础原理了解AI的运作模式及WordPress如何与AI交互集成。
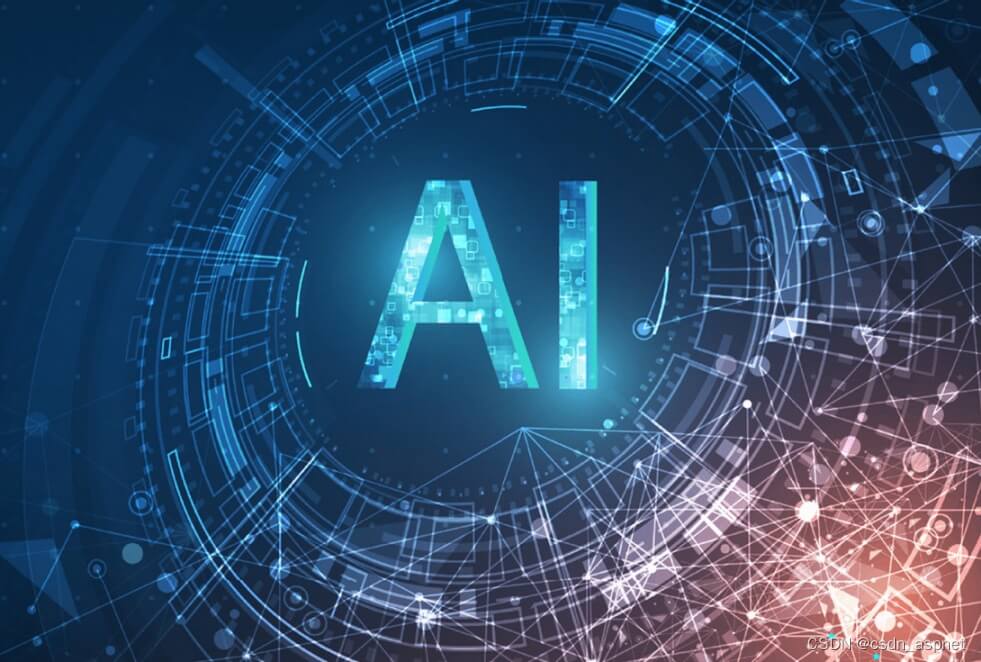
PHP 主要用于服务器端脚本和 Web 开发,虽然它不是构建复杂 AI 模型的首选语言,但借助现有的机器学习库和工具,PHP 仍然可以实现一些简单的 AI 功能。以下是几种在 PHP 中实现 AI 模型的方法:
1. 使用现成的机器学习服务
a. 使用 TensorFlow Serving 或其他 RESTful API 服务
你可以使用 Python 等更适合机器学习的编程语言训练模型,并将其部署为 RESTful API 服务。然后,PHP 可以通过 HTTP 请求与该服务通信,进行预测或推理。
步骤:
- 训练模型:使用 Python 和 TensorFlow、PyTorch 等框架训练你的机器学习模型。
- 部署服务:使用 TensorFlow Serving、Flask、FastAPI 等工具将模型部署为 REST API。
- PHP 调用:在 PHP 中使用
cURL
或其他 HTTP 客户端库调用该 API 进行预测。
示例代码(PHP 调用 REST API):
<?php
$url = "http://localhost:8501/v1/models/my_model:predict";
$data = json_encode([
"instances" => [
["feature1" => 5.1, "feature2" => 3.5],
["feature1" => 4.9, "feature2" => 3.0]
]
]);
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $data);
curl_setopt($ch, CURLOPT_HTTPHEADER, ['Content-Type: application/json']);
$response = curl_exec($ch);
curl_close($ch);
$result = json_decode($response, true);
print_r($result);
?>
b. 使用 PHP 扩展或库
虽然 PHP 本身对机器学习的支持有限,但有一些扩展和库可以辅助实现简单的 AI 功能。
i. PHP-ML
PHP-ML 是一个用于 PHP 的机器学习库,提供了基本的机器学习算法和工具。
安装:
使用 Composer 安装 PHP-ML:
composer require php-ai/php-ml
示例代码(线性回归):
<?php
require 'vendor/autoload.php';
use Phpml\Regression\LeastSquares;
// 训练数据
$points = [
[1, 3],
[2, 5],
[3, 7],
[4, 9]
];
// 分离特征和目标
$X = array_column($points, 0);
$y = array_column($points, 1);
// 创建并训练模型
$model = new LeastSquares();
$model->train($X, $y);
// 预测
$prediction = $model->predict([5]);
echo "预测值: " . $prediction[0];
?>
ii. TensorFlow PHP API
TensorFlow PHP API 允许在 PHP 中使用 TensorFlow 模型。不过,使用起来相对复杂,且性能可能不如原生 Python 接口。
安装:
需要编译 TensorFlow C 库并安装 PHP 扩展,具体步骤请参考 官方文档。
示例代码:
<?php
// 加载模型
$model = new TF_Model('path/to/saved_model');
// 进行预测
$input = [1.0, 2.0, 3.0];
$output = $model->predict($input);
print_r($output);
?>
2. 集成第三方 AI 服务
你可以利用第三方提供的 AI 服务,如 Google Cloud AI、AWS SageMaker、Azure Machine Learning 等,通过它们的 API 在 PHP 中调用这些服务进行各种 AI 任务,如图像识别、自然语言处理等。
示例(使用 Google Cloud Vision API):
<?php
require 'vendor/autoload.php';
use Google\Cloud\Vision\V1\ImageAnnotatorClient;
putenv('GOOGLE_APPLICATION_CREDENTIALS=path/to/your/service-account-file.json');
$annotator = new ImageAnnotatorClient();
$image = file_get_contents('path/to/image.jpg');
$response = $annotator->labelDetection($image);
$labels = $response->getLabelAnnotations();
foreach ($labels as $label) {
echo $label->getDescription() . "\n";
}
$annotator->close();
?>
3. 使用命令行工具与 PHP 结合
你可以在服务器上使用 Python 等语言编写 AI 模型,并通过 PHP 调用命令行脚本来执行预测任务。
示例:
- Python 脚本(predict.py):
import sys
import json
from sklearn.linear_model import LinearRegression
import joblib
# 加载模型
model = joblib.load('model.pkl')
# 获取输入数据
data = json.loads(sys.stdin.read())
X = [[item['feature']] for item in data['instances']]
predictions = model.predict(X)
# 输出结果
print(json.dumps({"predictions": predictions.tolist()}))
- PHP 调用脚本:
<?php
$input = json_encode([
"instances" => [
["feature" => 5],
["feature" => 6]
]
]);
$command = "python3 predict.py";
$descriptorspec = [
0 => ["pipe", "r"], // 标准输入
1 => ["pipe", "w"], // 标准输出
2 => ["pipe", "w"] // 标准错误
];
$process = proc_open($command, $descriptorspec, $pipes);
if (is_resource($process)) {
fwrite($pipes[0], $input);
fclose($pipes[0]);
$output = stream_get_contents($pipes[1]);
fclose($pipes[1]);
$return_value = proc_close($process);
$result = json_decode($output, true);
print_r($result);
}
?>
4. 注意事项
- 性能与扩展性:PHP 在处理大量计算任务时性能有限,复杂的 AI 模型训练和推理可能不适合在 PHP 环境中完成。
- 开发效率:使用专门的机器学习语言(如 Python)和工具可以显著提高开发效率和模型性能。
- 安全性:在集成外部服务或调用命令行工具时,注意数据传输和存储的安全性,防止潜在的安全漏洞。
总结
虽然 PHP 不是实现复杂 AI 模型的最佳选择,但通过集成现成的机器学习服务、使用专门的 PHP 库或与命令行工具结合,PHP 仍然可以在一定程度上实现简单的 AI 功能。对于需要高性能和复杂模型的应用场景,建议使用更适合的编程语言和工具,并通过 API 等方式与 PHP 应用进行交互。
评论
抢沙发请登录后发表评论
社交账号登录